15.3. Linking CSS to HTML¶
To use CSS, we need to apply our style rules to a set of HTML code. There are three different places to add CSS in an HTML file:
Inline: The CSS gets placed inside individual HTML tags. This is a good place to add some quick, specific styling to a small number of elements.
There is no selector in inline CSS. Instead, the
style
attribute is used. With inline CSS, the styling rule only applies to that one HTML element.<tag style="declaration block">Content...</tag>
Internal: One or more CSS style rules are placed within the
head
element at the start of the HTML document. The<style>
tags wrap the CSS content.Internal CSS works great when we have a small number of style rules that we need to apply to the whole document. The general syntax is:
3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
<head> <title>My Web Page</title> <style> selector { property: value; property: value; /* Other property assignments */ } selector { property: value; property: value; } /* Other CSS rules */ </style> </head>
Note that the syntax for the CSS rules follows the format we used on the previous page.
External: The CSS style rules are placed in a separate file. This document only contains CSS code, and it is saved with a
.css
extension. The external CSS file must be linked to the HTML document in thehead
element.External CSS is great when we have large amounts of rules that apply to the whole page. We can also reuse an external CSS document by linking it to multiple webpages. The general syntax for this is:
1 2 3 4
<head> <title>My Web Page</title> <link rel="stylesheet" type="text/css" href="style.css"> </head>
Let’s step through what’s going on in line 3:
<link>
is the HTML tag that tells the browser to connect a webpage and a separate, named file.rel
describes how the link relates to the page. In this case, the linked file provides style instructions, so we setrel
to the value"stylesheet"
.type
tells the browser what kind of content is saved within the linked file.type
should be set to"text/css"
for all style sheets. This tells the browser to expect CSS syntax when it opens the file.href
provides the path to the CSS document as well as its name. The path tells the browser where to look for the file it needs to open.In this case,
href="style.css"
tells the browser, “Look in the same folder as the HTML file, and open the document calledstyle.css
.”Note
The
href
path can describe where a file is stored on a computer, or it can be a web address where the CSS code can be accessed.
15.3.1. CSS Order of Importance¶
Since CSS instructions can be placed in three locations, it’s not hard to create conflicting style rules. If an external CSS file sets a heading text to blue, while an internal rule sets the color to red, which one wins? To deal with cases like this, browsers follow a specific order when applying styles.
Inline CSS receives the highest importance. It overrules any other styling.
Internal CSS comes next in importance.
External CSS receives the lowest level of importance.
The different CSS selectors also follow a specific order:
id
styling rules receive higher importance.class
styling rules come next.element
level styling rules receive the lowest importance.
Finally, nesting HTML elements inside others affects their styling. Any rules for the internal element override the rules on the outer element.
Example
The following HTML code nests 3 section
elements. Note how the style
rules for the inner elements get applied instead of the outermost color
choice.
1 2 3 4 5 6 7 8 9 | <section style="background-color:lightblue">
Outer section...
<section style="background-color:yellow">
Inner section...
<section style="background-color:white">Innermost section...</section>
Inner section...
</section>
Outer section...
</section>
|
Output
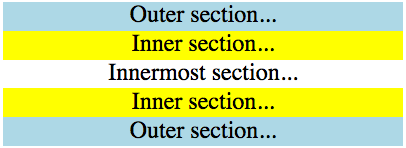
Tip
This order of importance is actually quite useful. We use it to our advantage whenever we need to override a more general style rule with one designed for a specific element.
15.3.2. Try It!¶
The editor below contains some plain HTML and a style.css
file. Follow the
instructions below the code to practice adding CSS style rules to the document.
Also, pay attention to how the order of importance affects how the rules get
applied.
On line 7, use the
<link>
tag to connect thestyle.css
file to the HTML code. Properly done, you will see the plain webpage change quite a bit. This is an example of bringing in external CSS.Note that the
li
selector fromstyle.css
affects all list elements on the page. On line 15, add BOTHclass="small-red-text"
andid="large-blue-item"
to the<li>
tag. Which style rule gets applied (element
,class
, orid
)?Inside
<head></head>
and below the<link>
tag, add the following code. This is an example of internal CSS:8 9 10 11 12 13
<style> li { color: green; text-decoration: underline wavy; } </style>
What level of importance does the internal
li
selector receive compared to the externalli
,class
, andid
rules?Add a
section
selector to the internal CSS. Set the background to a color of your choice. Does this internal rule override the font, text color, and border rules set instyle.css
?Use inline styling to add a border to one of the
<section>
tags. One fun option isstyle="border:4px double blue"
.4px
sets the border thickness to 4 pixels.double
refers to the border type (solid
,dashed
, anddotted
are also options).Does this inline CSS affect the
section
properties set by the internal and external style rules?
15.3.3. Check Your Understanding¶
Question
What is the order of importance for CSS style rules?
- Internal > External > Inline
- Inline > Internal > External
- Inline > External > Internal
- External > Internal > Inline
Question
What is the order of importance for CSS selectors?
- element > class > id
- element > id > class
- class > id > element
- class > element > id
- id > element > class
- id > class > element
Question
In the head
element of an HTML file, an internal CSS rule sets the text
color for all p
elements to blue. The HTML file also links to external
CSS that defines an id
selector to make red text (#red-text
).
Given the following HTML code, what color will the text be on the screen?
<p id="red-text" style="color: yellow">This is some text...</p>
- red
- blue
- yellow
- orange