28.8. Studio: TypeScript¶
Let's practice TypeScript by creating classes for rocket cargo calculations.
28.8.1. Starter Code¶
If you have not already done so, follow the instructions given in the TypeScript exercises to fork the GitHub repository.
Use the terminal to check that you are in the master
branch, then navigate
into the studio
folder.
$ git branch
* master
solutions
$ pwd
/typescript-lc101-projects
$ ls
exercises studio
$ cd studio
$ ls
index.ts Payload.ts
From the file tree in VSCode, open the index.ts
file.
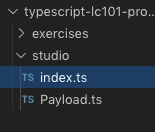
28.8.2. Requirements¶
Create classes for
Astronaut
,Cargo
, andRocket
. (Details below).All classes should be defined in their own files.
Use the new classes to run a simulation in the
index.ts
file.
In the starter code, you will notice that an interface named Payload
has
been declared. This interface ensures that any class that implements it will
have a massKg
property.
28.8.3. Classes¶
Create three new files---
Astronaut.ts
,Cargo.ts
, andRocket.ts
. To do this in VSCode, click the "New File" button and enter the file name. Another option is to run the commandtouch new_file_name
in the terminal.Define each class (
Astronaut
,Cargo
,Rocket
) in a separate file. Each class should be exported usingexport
.export class Astronaut { // properties and methods }
As needed, the classes can be imported using
import
.import { Astronaut } from './Astronaut';
28.8.3.1. Astronaut Class¶
Defined in
Astronaut.ts
Implements the
Payload
interfaceProperties
massKg
should be a number.name
should be a string.
Constructor
Parameter
massKg
should be a number.Parameter
name
should be string.Sets value of
this.massKg
andthis.name
.
28.8.3.2. Cargo Class¶
Defined in
Cargo.ts
Implements the
Payload
interfaceProperties
massKg
should be a number.material
should be a string.
Constructor
Parameter
massKg
should be a number.Parameter
material
should be a string.Sets value of
this.massKg
andthis.material
28.8.3.3. Rocket Class¶
Defined in
Rocket.ts
.Properties:
name
should be a string.totalCapacityKg
should be a number.cargoItems
should be an array ofCargo
objects.Should be initialized to an empty array
[]
astronauts
should be an array ofAstronaut
objects.Should be initialized to an empty array
[]
Constructor:
Parameter
name
should be a string.Parameter
totalCapacityKg
should be a number.Sets value of
this.name
andthis.totalCapacityKg
Methods:
sumMass( items: Payload[] ): number
Returns the sum of all
items
using each item'smassKg
property
currentMassKg(): number
Uses
this.sumMass
to return the combined mass ofthis.astronauts
andthis.cargoItems
canAdd(item: Payload): boolean
Returns
true
ifthis.currentMassKg() + item.massKg <= this.totalCapacityKg
addCargo(cargo: Cargo): boolean
Uses
this.canAdd()
to see if another item can be added.If
true
, addscargo
tothis.cargoItems
and returnstrue
.If
false
, returnsfalse
.
addAstronaut(astronaut: Astronaut): boolean
Uses
this.canAdd()
to see if another astronaut can be added.If
true
, addsastronaut
tothis.astronauts
and returnstrue
.If
false
, returnsfalse
.
28.8.4. Simulation in index.ts
¶
Paste the code shown below into index.ts
.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | import { Astronaut } from './Astronaut';
import { Cargo } from './Cargo';
import { Rocket } from './Rocket';
let falcon9: Rocket = new Rocket('Falcon 9', 7500);
let astronauts: Astronaut[] = [
new Astronaut(75, 'Mae'),
new Astronaut(81, 'Sally'),
new Astronaut(99, 'Charles')
];
for (let i = 0; i < astronauts.length; i++) {
let astronaut = astronauts[i];
let status = '';
if (falcon9.addAstronaut(astronaut)) {
status = "On board";
} else {
status = "Not on board";
}
console.log(`${astronaut.name}: ${status}`);
}
let cargo: Cargo[] = [
new Cargo(3107.39, "Satellite"),
new Cargo(1000.39, "Space Probe"),
new Cargo(753, "Water"),
new Cargo(541, "Food"),
new Cargo(2107.39, "Tesla Roadster"),
];
for (let i = 0; i < cargo.length; i++) {
let c = cargo[i];
let loaded = '';
if (falcon9.addCargo(c)) {
loaded = "Loaded"
} else {
loaded = "Not loaded"
}
console.log(`${c.material}: ${loaded}`);
}
console.log(`Final cargo and astronaut mass: ${falcon9.currentMassKg()} kg.`);
|
28.8.5. Compile and Run index.ts
¶
Use the terminal in VSCode to compile your
index.ts
file. This will also compile the modules you imported into the file (Astronaut.ts
,Rocket.ts
, etc.).Use the command
node index.js
to run the JavaScript file created during the build process.
$ ls
Astronaut.ts Cargo.ts Payload.ts Rocket.ts index.ts
$ tsc index.ts
$ ls
Astronaut.js Cargo.js Payload.js Rocket.js index.js
Astronaut.ts Cargo.ts Payload.ts Rocket.ts index.ts
$ node index.js
28.8.5.1. Expected Console Output¶
Mae: On board
Sally: On board
Charles: On board
Satellite: Loaded
Space Probe: Loaded
Water: Loaded
Food: Loaded
Tesla Roadster: Not loaded
Final cargo and astronaut mass: 5656.78 kg.
28.8.6. Submitting Your Work¶
Once you have your project working, use the terminal to commit and push your changes up to your forked GitHub repository.
Login to your account and navigate to your project. Copy the URL.
In Canvas, open the TypeScript studio assignment and click the "Submit" button. An input box will appear.
Paste the URL for your GitHub project into the box, then click "Submit" again.