28.7. Exercises: TypeScript¶
28.7.1. Part 0 - Get the Starter Code¶
Login to your GitHub account.
Fork the typescript-lc101-projects repository.
Use the terminal to clone your fork from GitHub. If you need a reminder for how to do this, refer to the Git studio.
Use the terminal to navigate into the
typescript-lc101-projects
folder, then into theexercises
subfolder.$ ls typescript-lc101-projects $ cd typescript-lc101-projects $ ls exercises studio $ cd exercises $ ls SpaceLocation.ts parts1-5.ts tsconfig.json
28.7.2. Part 1 - Declare Variables With Type¶
Run VSCode and open the typescript-lc101-projects
folder. From the file
tree, select the parts1-5.ts
file.
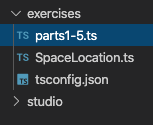
VSCode file tree¶
In the space indicated, declare and assign a variable for each of the following:
Variable Name |
Type |
Value |
---|---|---|
spacecraftName |
string |
|
speedMph |
number |
17500 |
kilometersToMars |
number |
225000000 |
kilometersToTheMoon |
number |
384400 |
milesPerKilometer |
number |
0.621 |
28.7.3. Part 2 - Print Days to Mars¶
In the same file you opened in Part 1, do the following.
Declare and assign these variables.
Remember: variable declarations in TypeScript include the type!
milesToMars
is a number with the value ofkilometersToMars * milesPerKilometer
.hoursToMars
is a number with the value ofmilesToMars / speedMph
.daysToMars
is a number with the value ofhoursToMars / 24
.
Write a
console.log
statement that prints out the days to Mars.Use template literal syntax and the variables
${spacecraftName}
and${daysToMars}
.
Use the terminal in VSCode to compile your
.ts
file, then use the commandnode parts1-5.js
to run the JavaScript file created during the build process.
Terminal
$ tsc parts1-5.ts
$ node parts1-5.js
Determination would take 332.67857142857144 days to get to Mars.
28.7.4. Part 3 - Create a Function¶
In the space indicated, define a function that calculates the days it would take to travel to a location.
Function name
getDaysToLocation
Parameter
kilometersAway
must be a number.
Returns the number of days to a location.
Use the same calculations as in Part 2.1.
Inside the function, make the variable names generic. Use
milesAway
andhoursToLocation
instead ofmilesToMars
andhoursToMars
.The function should declare that it returns a
number
.
Print out the days to Mars.
Move the output statement from part 2 below your new function.
Refactor the template literal to use
${getDaysToLocation(kilometersToMars)}
and${spacecraftName}
.
Print out the days to the Moon.
Add another output statement and template literal using
${getDaysToLocation(kilometersToTheMoon)}
and${spacecraftName}
.
Use the terminal in VSCode to recompile your
.ts
file, then run theparts1-5.js
file again.
Terminal
$ tsc parts1-5.ts
$ node parts1-5.js
Determination would take 332.67857142857144 days to get to Mars.
Determination would take 0.5683628571428571 days to get to the Moon.
28.7.5. Part 4 - Create a Spacecraft Class¶
Organize getDaysToLocation
and the variables for name, speed, and miles per
kilometer by moving them into a class.
Define a class named
Spacecraft
.Properties
milesPerKilometer: number = 0.621;
name: string;
speedMph: number;
Constructor
name
is the first parameter and it MUST be a string.speedMph
is the second parameter and it MUST be a number.Sets the class properties using
this.name
andthis.speedMph
.
Note
Once you complete the constructor, be sure to remove the variables you defined in part 1 (
spacecraftName
,milesPerKilometer
, andspeedMph
.Move the function
getDaysToLocation
, defined in Part 3, into theSpacecraft
class.Remember to place the function after the constructor.
Update the function to reference the class properties
this.milesPerKilometer
andthis.speedMph
.
Create an instance of the
Spacecraft
class.let spaceShuttle = new Spacecraft('Determination', 17500);
Print out the days to Mars.
Use template literals,
${spaceShuttle.getDaysToLocation(kilometersToMars)}
and${spaceShuttle.name}
.
Print out the days to the Moon.
Use template literals,
${spaceShuttle.getDaysToLocation(kilometersToTheMoon)}
and${spaceShuttle.name}
.
Use the terminal in VSCode to recompile your
.ts
file, then run the.js
file again.
Terminal
$ tsc parts1-5.ts
$ node parts1-5.js
Determination would take 332.67857142857144 days to get to Mars.
Determination would take 0.5683628571428571 days to get to the Moon.
28.7.6. Part 5 - Export and Import the SpaceLocation Class¶
From the file tree in VSCode, open the
SpaceLocation.ts
file.Paste in the code provided below.
Notice the
export
keyword. That is what allows us to import it later.
1 2 3 4 5 6 7 8 9
export class SpaceLocation { kilometersAway: number; name: string; constructor(name: string, kilometersAway: number) { this.name = name; this.kilometersAway = kilometersAway; } }
Add the function
printDaysToLocation
to theSpacecraft
class.Notice that it takes a parameter of type
SpaceLocation
.
1 2 3
printDaysToLocation(location: SpaceLocation) { console.log(`${this.name} would take ${this.getDaysToLocation(location.kilometersAway)} days to get to ${location.name}.`); }
Import
SpaceLocation
intoparts1-5.ts
.Paste
import { SpaceLocation } from './SpaceLocation';
to the top ofparts1-5.ts
.
Replace the earlier
console.log
statements by using the class instance to print out the days to Mars and the Moon.47 48
spaceShuttle.printDaysToLocation(new SpaceLocation('Mars', kilometersToMars)); spaceShuttle.printDaysToLocation(new SpaceLocation('the Moon', kilometersToTheMoon));
Use the terminal in VSCode to compile your
.ts
file, then run the.js
file again.
Terminal
$ tsc parts1-5.ts
$ node parts1-5.js
Determination would take 332.67857142857144 days to get to Mars.
Determination would take 0.5683628571428571 days to get to the Moon.
28.7.7. Sanity Check¶
The typescript-lc101-projects
repository has two branches---master
and
solutions
. 'Nuff said.