11.3. The random
Module¶
We often want to use random numbers in programs. Here are a few typical uses:
To play a game where the computer needs to throw some dice, pick a number, or flip a coin.
To select a random item from a list.
To randomly choose an event to apply to a virtual city.
To encrypt your banking session on the Internet.
Python provides a module called random
that helps with tasks like this.
11.3.1. Generate a Random Number¶
Press the Run button a number of times for the program below. Note that the printed values change each time. These are random numbers.
The random() function returns a float value in the range 0.0 - 1.0
(including 0.0 but not 1.0). If we need a larger float result,
say from 0.0 to 10.0, we simply multiply the result from random()
by the
top value we want.
In the case shown below, we’ve converted the result from random()
to a
number in the range 0.00 - 4.99. Press the Run button several times to
confirm the results, then try changing line 4 to produce a different range.
What happens if we change the multiplier?
What happens if we add a number to
random.random()
instead of multiplying?What if we multiply
random.random()
by a value and then add a number to the result?
The randint() function is a useful modification of random()
. It
generates an integer between the two arguments. In this case, however, the
results include both end points.
Run this program several times to check the behavior of the randint()
function.
Try changing the arguments inside the ()
to see how that affects the
numbers produced.
11.3.1.1. Not Really Random¶
It is important to note that random number generators are based on algorithms. This means that the results are predictable and not truly random.
Each algorithm starts with a seed value, which the code uses to create a result. Every time we ask for another random number, we get one based on the current seed, and the value of the seed gets updated. If we figure out how the seed values are generated, then we can predict what the next “random” result will be.
The good news is that each time we run the program, the seed value is likely to be different (for example, the number of seconds since midnight). This means that even though the random numbers come from following an algorithm, we will likely get random behavior each time we run our program.
Note
Theoretically, if you could figure out the algorithm and seed values for a lottery game, then you could always pick the winning numbers!
Doing this would be really, really hard, however.
11.3.2. Select an Item from a Collection¶
Another useful function from the random
module is the choice()
function. It selects a random item from a string, list, or other collection.
Run the following program several times to see how this works:
We could do the same thing with randint()
and bracket notation, but the
choice()
function wraps this up into a simple shortcut.
1 2 3 4 5 6 7 8 9 | import random
colors = ['red', 'orange', 'yellow', 'green', 'blue', 'indigo', 'violet']
# Select a random integer from 0 - 6:
index = random.randint(0, len(colors)-1)
# Save the random element from the list:
color_choice = colors[index]
|
Try It!
Randomly change the color of the turtle before it draws each side of the polygon!
Add the statement bob.color(random.choice(colors))
to the code below.
11.3.3. Random Turtle Walk¶
Let’s have a little more turtle fun!
Up until now, we have always given a specific direction (left
or right
)
and angle whenever we turn a turtle. Lets throw in some random values to make
the path our turtle follows less predictable.
Example
Currently, the turtle takes 10 steps, and it always turns right by 90 degrees. Run the program first to see this behavior.
Now make the following changes:
At the start of the loop, define a
coin_toss
variable and assign it the result ofrandom.randint(0,1)
. As the name of the variable tells us, we can treat this like a coin toss, with0
standing for heads, and1
standing for tails.If you don’t like working with 0 and 1, you can use
random.choice('heads', 'tails')
instead. However, numbers are easier to work with, and you are less likely to mistype 0 and 1.Place the turn command inside a conditional as follows:
14 15 16 17
if coin_toss == 0: bob.right(90) else: bob.left(90)
Now
bob
turns left or right depending on the random choice of0
or1
. Run the program several times and compare the paths.Next, let’s make
bob
rotate by a random number of degrees. Replace the argument90
withrandom.randint(0, 180)
. Run the program several times to see the result.Finally, randomly assign the number of steps
bob
takes to a value between 5 and 25. Run the program several times to check your work.
Here are some bonus tasks for you to try as well:
Add a
colors
list and randomly assign a color to the turtle before each line is drawn.Randomly select the length of each line drawn.
Randomly change the speed before or during the turtle’s walk.
Move the walking code into a
random_walk
function that takes a turtle and the number of steps as parameters. Callrandom_walk
to makebob
move.Add a second turtle and have it take a stroll as well.
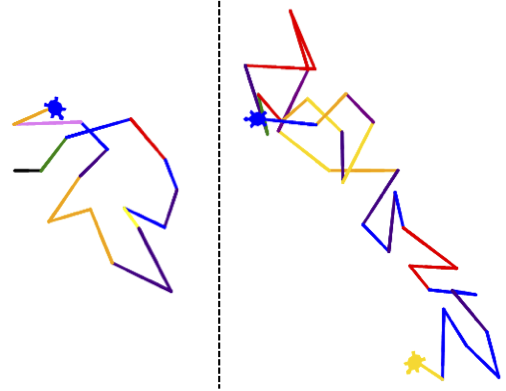
A single, multi-color turtle path. Two multi-color paths drawn by different turtles.¶
11.3.4. Check Your Understanding¶
Question
Which of the following shows the correct code to return the result of rolling a 6-sided dice?
- random.randint(0, 7)
- random.randint(1, 7)
- random.randint(0, 6)
- random.randint(1, 6)
Question
One reason that lotteries don’t use computers to pick the winning numbers is:
- There is no computer on the stage for the drawing.
- Computers don’t really generate random numbers.
- Computers would generate the same numbers for each drawing.
- The computer can’t tell what values were already selected, so it might repeat the same number several times.