8.1. List Basics¶
The Python list data type is another example of an ordered collection. Lists store data values, which are called elements. Just like with strings, each element has its own index value. However, strings are ordered collections of characters and the elements of lists can be of any data type.
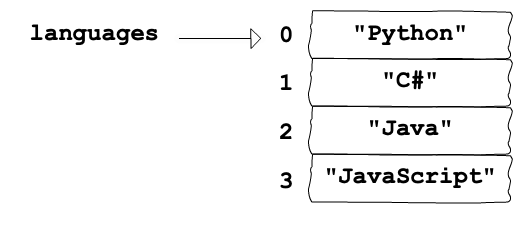
This list contains four strings, and each value has its own index position.¶
8.1.1. Create a New List¶
There are several ways to create a new list. The simplest is to enclose the
elements in square brackets []
, with each element separated from the others
by commas.
Example
1 2 3 4 | numbers = [5, 15, 15, 0, 25]
strings = ['banana', 'broccoli', 'kale', 'applesauce']
mixed_data = ["Hello", 42, True, 3.14, [-3, 48.5]]
empty_list = []
|
Line 1 assigns a list of five integers to the variable
numbers
.Line 2 assigns a list of four strings.
The elements of a list don’t have to be the same data type! The list in line 3 contains a string, an integer, a boolean, a float, and another list.
Line 4 assigns a special list that contains no elements, called the empty list.
Note
A list within another list is said to be nested. We will explore this idea later in the chapter.
8.1.2. Accessing Elements¶
With strings, we accessed individual characters by using square brackets. With lists, we use square brackets to access list elements. The integer or expression inside the brackets gives the index for the element we want.
Remember that index values start at 0.
Any integer (or an expression that returns a whole number) can be used as the
index. Negative index values identify elements from right-to-left, beginning
at -1
for the last element in the list.
Try It!
Use index values to print out different elements from a list.
Add at least three new elements to
my_list
. Feel free to use values of the same data type or different data types.Note that line 4 prints the entire list (with brackets) to the console.
Change the index value in line 5 to print different elements from
my_list
. Be sure to try both positive and negative integers.Try using an expression (like
len(my_list) - 2
) inside the brackets in line 5.What happens if you use an index value larger than the number of elements in the list?
8.1.3. Check Your Understanding¶
Question
List elements must all be the same data type.
- True
- False
Question
Identify the length of these two lists. (The answers list classes
first,
then teachers
).
1 2 | classes = ["Chemistry, US History, Intro To Coding"]
teachers = ["Cortez", "Holmes", "Bracey"]
|
- 1 and 3
- 3 and 1
- 3 and 3
- 1 and 1
Question
Identify the output from the following statements:
1 2 | a_list = ["Hello", 42, True, 3.14]
print(a_list[2])
|
- Hello
- 42
- True
- 3.14