4.3. Variables¶
One of the most powerful features of a programming language is the ability to use variables. A variable is a name that refers to a value. Recall that a value is a single, specific piece of data, such as a specific number or string. Variables allow us to store values for later use.
You can think of a variable as a label that points to a piece of data.
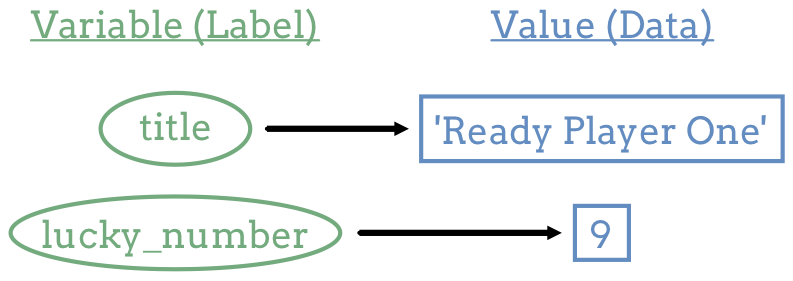
The variable title
points to the string value 'Ready Player One'
, and lucky_number
points to the integer value 9
.¶
With this picture in mind, let’s learn how to create and use variables in Python.
4.3.1. Assigning Values¶
Assignment statements create new variables and also give them values to refer to. The syntax for an assignment statement is:
variable_name = value
The assignment statement links a name, on the left hand side of the =
, with
the value on the right hand side. The value refers to any type of data.
Example
message = "Python ROCKS!"
num = 17
pi = 3.14159
This example makes three assignments. The first assigns the string value
"Python ROCKS!"
to a new variable named message
. The second gives the
integer 17
to num
, and the third assigns the float 3.14159
to a
variable called pi
.
Warning
The =
sign does NOT work both ways. 17 = num
causes an error. The
variable name MUST be on the left, and the value MUST be on the right.
One common mistake is to confuse the assignment operator =
with the idea of
equality (things being the same). Equality compares two values, like 5
vs. 4
or 'dog'
vs. 'dog'
. Assignment gives a value to a variable.
We will explore equality later and see that it uses the ==
operator
instead of =
.
4.3.2. Evaluating Variables¶
Once we create a variable and assign it a value, we can use the variable name to refer to that value.
Example
These two examples give the exact same output.
print("Hello, World!")
1 2 | message = "Hello, World!"
print(message)
|
In the second example just above, the variable name message
points to the
value "Hello, World"
. print(message)
means the same thing as print("Hello, World!")
, so we
say that message
evaluates to "Hello, World!"
Example
1 2 3 4 5 6 7 | message = "Python ROCKS!"
num = 17
pi = 3.14159
print(message)
print(num)
print(pi)
|
Console Output
Python ROCKS!
17
3.14159
In each case, the printed result is the value of the variable.
4.3.2.1. Try This¶
Do variables have data types? Run the following code to find out.
The type of a variable is same as the data type of its current value.
4.3.3. Reassigning Variables¶
We use variables in a program to store values, like the current score at a football game. Just like a score, variables can change over time.
To see this, read and then run the following program. Notice how we change the
value of day
three times. We even give it a value of a different data type.
A great deal of programming involves asking the computer to remember things. For example, we might want to keep track of the number of missed calls on our phones. Each time we miss another call, we can update a variable to reflect the new total.
4.3.4. Check Your Understanding¶
Question
What is printed when the following code runs?
1 2 3 4 | day = "Thursday"
day = 32.5
day = 19
print(day)
|
- Thursday
- 32.5
- 19
- Thursday 32.5 19
Question
How can you determine the data type of a variable?
- Print out the value of the variable.
- Use
type()
. - Use it in a known equation and print the result.
- Look at the assignment of the variable in the code.