1.3. Java in the Terminal¶
1.3.1. Mac Users¶
Let’s write a simple “Hello, World” program and watch the JDK in action.
In the future, we’ll be doing most of our Java coding with the IntelliJ IDE. IntelliJ contains many features to help us write Java properly and easily, including its own compiler. For now though, we’ll use a simpler text editor so we can demonstrate what we get with the JDK.
In the text editor of your choice, create and save a file called
HelloWorld.java
and include the code below:
1 2 3 4 5 6 7 8 | public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World");
}
}
|
We’ll discuss the syntax of this program soon, but you can likely predict that this program has an expected output of “Hello, World”. To test this hypothesis, open a terminal window and navigate to the parent directory of your new file. Run:
java HelloWorld.java
You should see your greeting printed!
Recall from the walk-through on the previous page, Java needs to be compiled before executing. Java version 11 introduced
the capability to compile single-file Java programs without explicitly running a command to compile. If our
Hello, World
program were more complex and contained another file, we would need to first run
javac HelloWorld.java
, to compile, followed by java HelloWorld.java
.
1.3.2. Windows Users¶
Let’s write a simple “Hello, World” program and watch the JDK in action.
In the future, we’ll be doing most of our Java coding with the IntelliJ IDE. IntelliJ contains many features to help us write Java properly and easily, including its own compiler. For now though, we’ll use a simpler text editor so we can demonstrate what we get with the JDK.
In the text editor of your choice, create and save a file called
HelloWorld.java
and include the code below:
1 2 3 4 5 6 7 8 | public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World");
}
}
|
We’ll discuss the syntax of this program soon, but you can likely trust your gut that this program has an expected output of “Hello, World”.
Warning
Whenever using the terminal in this course, use Git Bash as opposed to Windows Command Prompt.
To test this hypothesis, open a terminal window and navigate to the parent directory of your new file.
In a separate window, navigate to the bin
folder in the Java Development Kit to get the file path (the image below shows you how to get there from the C: Drive). Copy the file path.
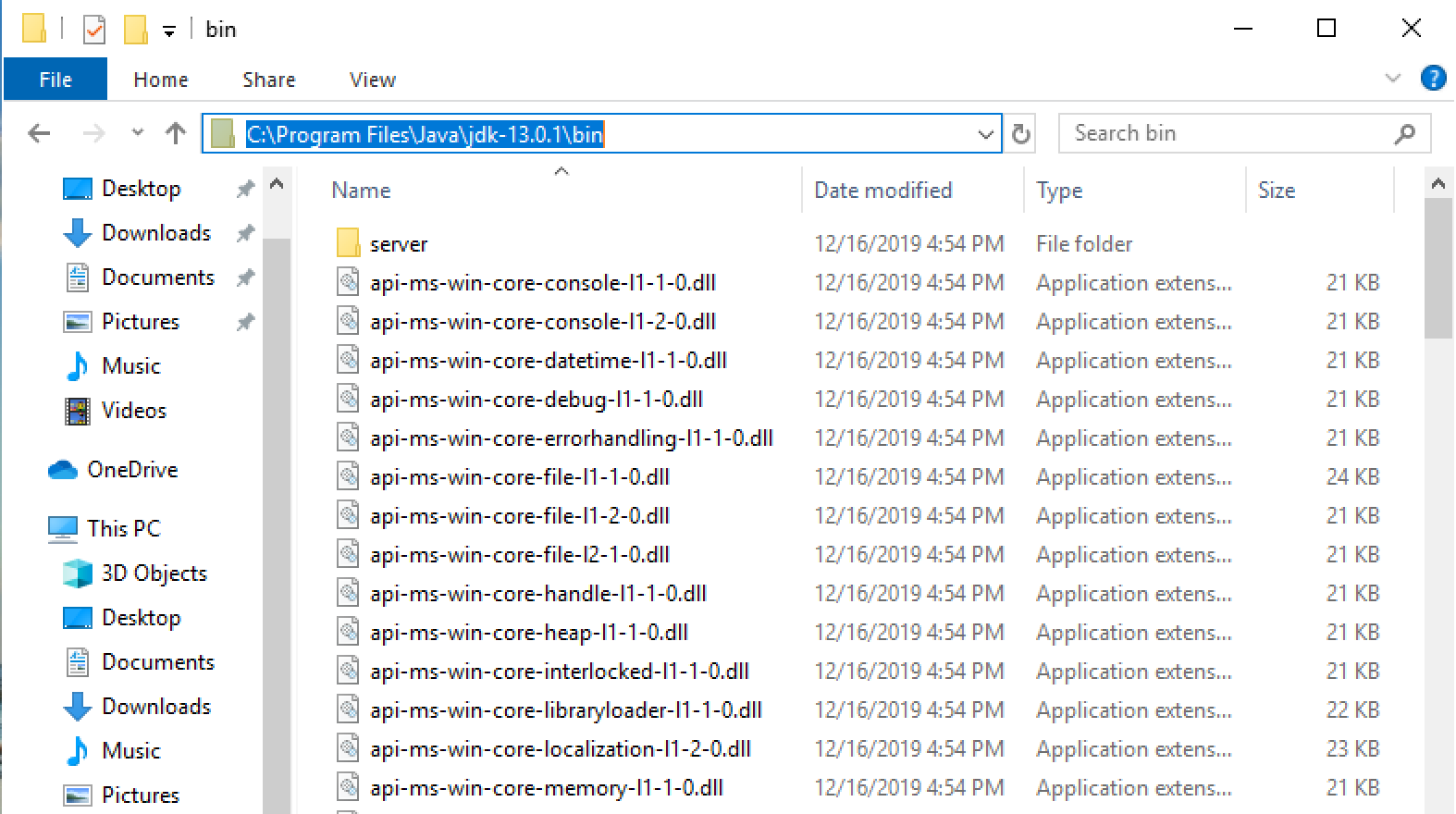
Run the following command, replacing the {filepath}
with the file path to your JDK that you just copied:
set PATH=%PATH%;{filepath}
This command sets a path in our system for java
so that we can compile and run Java programs.
To make use of the new java
command, you may have to restart your terminal window by closing it and opening a new one.
Make sure that you are in your project’s directory.
java HelloWorld.java
You should see your greeting printed!
Recall from the walk-through on the previous page, Java needs to be be compiled before executing. Java version 11 introduced
the capability to compile single-file Java programs without explicitly running a command to compile. If our
Hello, World
program were more complex and contained another file, we would need to first run
javac HelloWorld.java
, to compile, followed by java HelloWorld.java
.
Note
These steps change the path in just that directory. While this is sufficient to get us through the rest of the course, you may want change the system path for your whole system. Check out these instructions to change the path globally.