Task 1: Intro and Starter Code
This task puts your unit testing, modules making, and exception handling knowledge to use by writing tests and classes for the Mars rover named Curiosity.
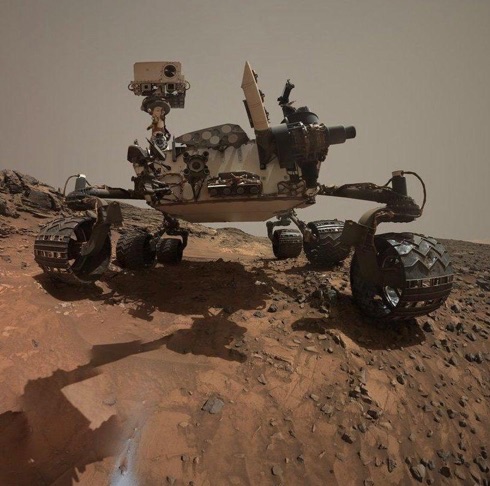
You will create a simulation for issuing commands to Curiosity. The idea is to create a command at mission control, convert that command into a message, send it to the rover, then have the rover respond to that message.
We will provide descriptions of the required features you need to implement in three separate classes:
- Command: A type of object containing a
commandType
property.commandType
is one of the given strings in the table below. SomecommandTypes
are coupled with avalue
property, but not all. EveryCommand
object is a single instruction to be delivered to the rover. - Message: A
Message
object has aname
and contains severalCommand
objects.Message
is responsible for bundling the commands from mission control and delivering them to the rover. - Rover: An object representing the mars rover. This class contains information on the rover’s
position
, operatingmode
, andgeneratorWatts
. It also contains a function,receiveMessage
that handles the various types of commands it receives and updates the rover’s properties.
In true TDD form, you will be asked to first write the appropriate unit tests for these features, then write the code in the given class to pass those tests.
Sections:
Get the Starter Code
Fork and Clone the following repository:Assignment #3: Mars Rover
How-To TDD
Recall that in TDD, you write the test for a given behavior before you code the actual function. Feel free to review the Test/Code cycle while you work on this project.
- Focus on one test at a time.
- Write the test and then create the code to make it pass.
- Only write the minimum amount of code needed to make the test pass.
- There are some constraints on how you can implement these features. A description of each class is below.
Each numbered item describes a test. You should use the given phrases as the test descriptions when creating your it
statements. You must create 13 tests for this assignment.
Do NOT try to write all of the tests at once. Doing so will be inefficient and will cause excessive frustration.
Once you are ready, move on to the next section: Command Class