Assignment #3: Mars Rover¶
This task puts your unit testing, modules making, and exception handling knowledge to use by writing tests and classes for the Mars rover named Curiosity.
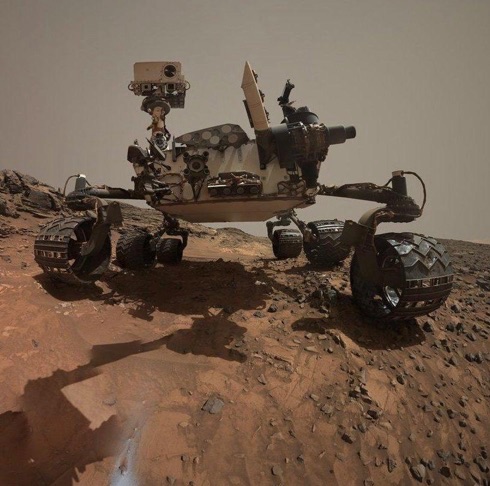
Selfie of Curiosity on Mars.¶
You will create a simulation for issuing commands to Curiosity. The idea is to create a command at mission control, convert that command into a message send it to the rover, then have the rover respond to that message.
We will provide descriptions of the required features you need to implement in three separate classes:
Command
: A type of object containing acommandType
property.commandType
is one of the given strings in the table below. SomecommandTypes
are coupled with avalue
property, but not all. EveryCommand
object is a single instruction to be delivered to the rover.Message
: AMessage
object has aname
and contains severalCommand
objects.Message
is responsible for bundling the commands from mission control and delivering them to the rover.Rover
: An object representing the mars rover. This class contains information on the rover'sposition
, operatingmode
, andgeneratorWatts
. It also contains a function,receiveMessage
that handles the various types of commands it receives and updates the rover's properties.
In true TDD form, you will be asked to first write the appropriate units tests for these features, then write the code in the given class to pass those tests.
Get the Starter Code¶
Fork this replit.
How-To TDD¶
Recall that in TDD, you write the test for a given behavior before you code the actual function. Feel free to review the Test/Code cycle while you work on this project.
Focus on one test at a time.
Write the test and then create the code to make it pass.
Only write the minimum amount of code needed to make the test pass.
There are some constraints on how you can implement these features. A description of each class is below.
Each numbered item describes a test. You should use the given phrases as the
test descriptions when creating your it
statements. You must create 13
tests for this assignment.
Warning
Do NOT try to write all of the tests at once. Doing so will be inefficient and will cause excessive frustration.
Command
¶
Command
Class Description¶
We'll follow TDD practices for the creation of Message
and Rover
, but for
this class, Command
, we've provided the functionality. Command
is already
written for you and you do not need to modify it to write passing tests. Open up and
examine the file command.js
.
This class builds an object with two properties.
constructor(commandType, value)
commandType
is a string that represents the type of command. We will go over the details of the types when we get to theRover
class and tests. At this time, note that a command type will be one of the following:'MODE_CHANGE'
,'MOVE'
, or'STATUS_CHECK'
.To peek ahead at the full functionality of these types, refer to Command Types table.
value
is a value related to the type of command.
Example
let modeCommand = new Command('MODE_CHANGE', 'LOW_POWER');
let moveCommand = new Command('MOVE', 12000);
'MODE_CHANGE'
and 'MOVE'
are passed in as the commandType
'LOW_POWER'
and 12000 are passed in as the value
. Different command
types require different kinds of values. 'STATUS_CHECK'
takes no value.
Don't worry about the mode options for now. To peek ahead, see Rover Modes table.
Now that we've gone over the class, let's check out the tests.
Command
Tests¶
To begin, open and examine spec/command.spec.js
. One test has been created for
you. When a user creates a new Command
object from the class, we want to make
sure they pass a command type as the first argument.
Test 1¶
Note that the test description reads, "throws error if a command type is NOT passed into the constructor as the first parameter".
So far, you have many used expectations to check for equality. In the chapter on exceptions, we shared an example of how we might use an expectation to check if an exception is thrown. Refer back to that example for guidance on the syntax.
Click "Run" to verify that the test passes. Next, comment out lines 4-6 in
command.js
. Click "Run" again to verify that the test fails (the expected error is not thrown when theCommand
class is called).Restore lines 4-6 to
throw Error("Command type required.");
.Change 'Command type required.' on line 9 in
command.spec.js
to 'Oops'. Click "Run" again to verify that the test fails (the error message did not match"Command type required."
).Restore line 9 to 'Command type required.'.
Test 2¶
Create a second Command
test using, "constructor sets command type" as the
description. This test checks that the constructor
in the Command
class correctly sets the commandType
property in the new object.
Without editing,
command.js
contains the correct code. Click "Run" to verify that the first and second tests both pass.You do not need to use
expect().toThrow()
.You may not need to know the specific types of commands to write this test.
Test 3¶
Code a third test using, "constructor sets a value passed in as the 2nd
argument" as the description. This test checks that the constructor
correctly sets the value
property in the new object.
You may not need to know a proper
value
in order to write this test.
Click "Run" to verify that all 3 command tests pass.
Note
As you move through the remaining instructions, the amount of guidance will decrease. Refer to your earlier, passing tests to help you construct new tests and passing code.
Great job, astronaut! When you are ready to keep going, check out Part 2!
Submitting Your Work¶
Once your Rover
class is completed and you have written 13 passing specs, you are ready to submit.
Tip
Make sure that you did not edit either studentgrading.spec.js
, grading.js
, or any file in the helpers
directory inside spec
.
Changes to these files could effect your grade.
In Canvas, open the Mars Rover assignment and click the "Submit" button. An input box will appear.
Copy the URL for your repl and paste it into the box, then click "Submit" again.